In the new era of software, ensuring performance
and stability
under heavy loads
is very paramount. Load testing
is a critical step in the software development lifecycle, as it helps identify performance bottlenecks and vulnerabilities. In this blog post, we will delve into how we can leverage Swagger/OpenAPI
specifications, a popular API documentation
standard, along with K6
, a powerful load testing tool
, to create effective load tests for .NET applications. We will provide a step-by-step guide on generating load tests from Swagger/OpenAPI definitions, demonstrate how to use K6 to simulate realistic user behavior, and share tips on analyzing and interpreting the results. By the end of this post, you will have the knowledge and tools to create comprehensive load tests for your .NET applications. Let's dive in and get started!
Swagger/OpenAPI
Swagger/OpenAPI is a widely used standard for documenting and describing RESTful APIs (Application Programming Interfaces). It provides a way to define the structure, endpoints, request/response payloads, and authentication mechanisms of an API in a machine-readable format. Swagger/OpenAPI specifications are typically written in YAML or JSON and serve as a contract that allows developers to understand, consume, and interact with APIs accurately and consistently.
K6
K6 is an open-source, developer-centric load testing tool used for testing the performance and scalability of APIs, microservices, and websites. It allows you to write performance tests in JavaScript and execute them from the command line or integrate them into your continuous integration (CI) or continuous delivery (CD) pipeline.
Installation
To install the K6 using Docker, we can execute the following command:
docker pull grafana/k6
Not: Another option for installing K6 is to follow the installation instructions provided in the official K6 documentation, which can be found at https://k6.io/docs/get-started/installation.
OpenAPI Generator CLI
The OpenAPI Generator CLI is a robust tool that enables developers to generate client libraries, server stubs, and API documentation from OpenAPI Specification files. If you prefer to use Docker for containerization and isolation, installing OpenAPI Generator CLI as a Docker container is a seamless option. In this blog post, we will guide you through the steps to install OpenAPI Generator CLI using Docker, empowering you to harness its capabilities in your API development workflow.
Installation
To install the OpenAPI Generator CLI using Docker, we can execute the following command:
docker pull openapitools/openapi-generator-cli
Create WebApi template sample with Swagger/OpenAPI
We can create a template with Swagger documentation using the following command:
dotnet new webapi -minimal -n k6-load-test-generator-sample
Generate load Tests with OpenAPI Generator CLI
The following step involves executing the command below to generate load test scripts
. This command utilizes a Linux Docker container to mount
our Swagger/OpenApi Url
or Path
to the designated location
where we want to save or load the test scripts. (Run this command in root of your project)
docker run --rm -v ${PWD}:/local openapitools/openapi-generator-cli generate --skip-validate-spec -i http://host.docker.internal:5000/swagger/v1/swagger.json -g k6 -o local/LoadTests/
- In the command below,
/local
refers to the root directory of our projects. - In our example, we use
Url
to mount Swagger/OpenApi, with our project running on port 5000.
After successfully running the command, a script.js
file will be generated in the LoadTests
directory, based on our Swagger/OpenApi
configuration. Thanks to the OpenAPI Generator CLI used in the above command, we will have basic load tests for all APIs at our disposal.
import http from 'k6/http'
import { group, check, sleep } from 'k6'
const BASE_URL = 'http://localhost:5000'
// Sleep duration between successive requests.
// You might want to edit the value of this variable or remove calls to the sleep function on the script.
const SLEEP_DURATION = 1
// Global variables should be initialized.
export default function () {
group('/weatherforecast', () => {
// Request No. 1: GetWeatherForecast
{
let url = BASE_URL + `/weatherforecast`
let request = http.get(url)
check(request, {
OK: (r) => r.status === 200,
})
}
})
}
Note: For detailed configuration information on our load test, you can refer to the K6 Documentation. The default scripts generated by K6 are basic, but can be enhanced manually for advanced usage.
Run Load Tests with K6
To execute our load test, navigate to the LoadTests
directory and enter the following command:
k6 run script.js
The outcome of the load tests will provide you with relevant information, such as the processing duration and other key details about the request. This data will give you valuable insights into the performance of your application under varying loads, enabling you to make informed decisions to optimize its performance.
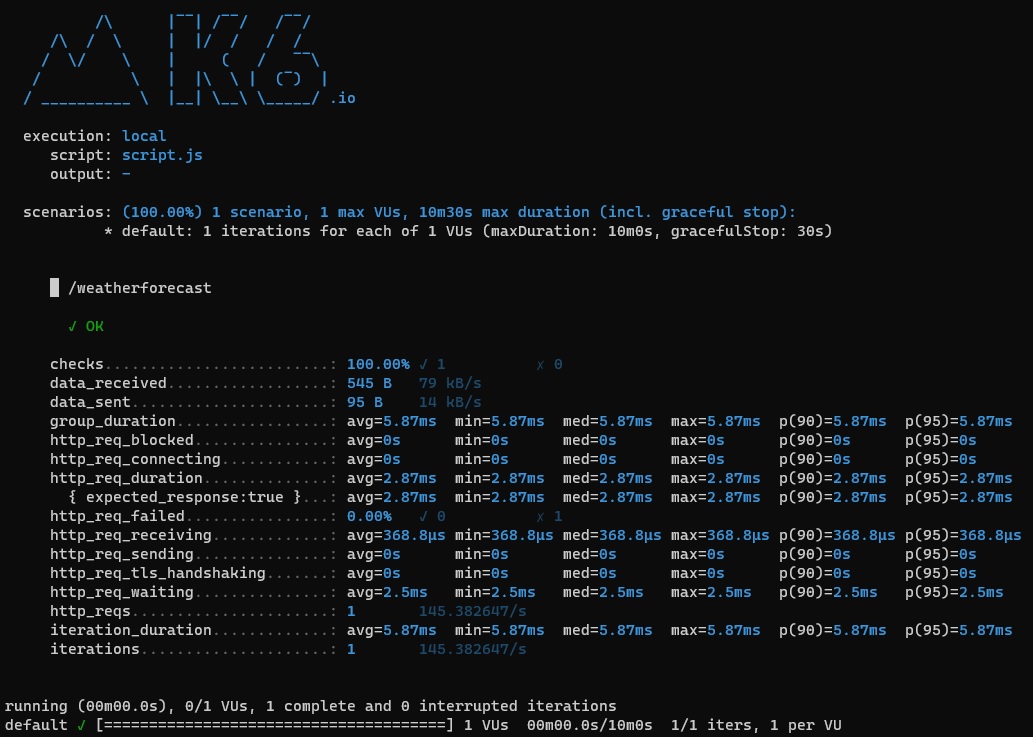
You can find the sample code in this repository:
🔗 https://github.com/meysamhadeli/blog-samples/tree/main/src/k6-load-test-generator-sample
Conclusion
Leveraging OpenAPI Generator CLI
in combination with K6
can greatly simplify and streamline the process of generating load tests
from Swagger/OpenAPI specifications
. The OpenAPI Generator CLI provides a comprehensive set of options for customizing the generation of test scripts in the K6 format, allowing developers to tailor their load tests to their specific requirements. This powerful combination allows for efficient load testing of APIs, helping to identify and address performance issues early in the development process. By automating the generation of load tests from Swagger/OpenAPI specifications, developers can save time and effort while ensuring that their APIs are thoroughly tested under realistic loads. Whether you're new to load testing or an experienced developer, incorporating OpenAPI Generator CLI with K6 into your API testing workflow can be a valuable tool for optimizing
the performance
and reliability
of your APIs.
Reference
https://k6.io/blog/load-testing-your-api-with-swagger-openapi-and-k6/